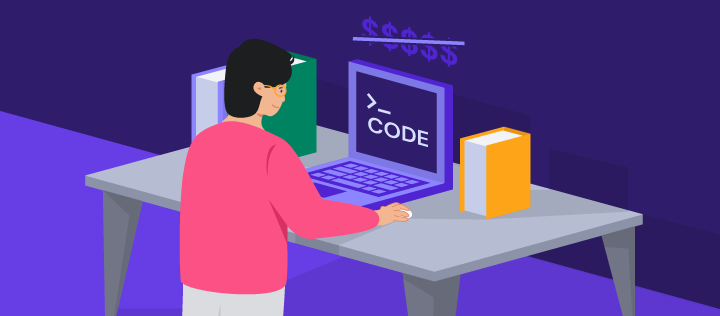
1. Understand the Problem
- Read Carefully: Take your time to read the problem statement thoroughly. Make sure you understand what is being asked.
- Identify Inputs and Outputs: Determine what the input is and what the expected output should be.
- Clarify Doubts: If anything is unclear, ask clarifying questions (in interviews) or re-read the problem to ensure you have all the necessary details.
- Edge Cases: Consider any edge cases that might be relevant, such as empty inputs, very large inputs, or special conditions.
2. Plan Your Approach
- Algorithm Selection: Decide on the algorithm or data structure that best suits the problem. This might involve recognizing patterns or similarities to problems you’ve solved before.
- Break It Down: Divide the problem into smaller, manageable parts. Outline the steps needed to solve each part.
- Pseudo Code: Write pseudo code to outline your approach. This helps in visualizing the logic without worrying about syntax.
3. Write the Code
- Start Simple: Begin by coding the basic structure of your solution. Focus on getting a simple version working first.
- Incremental Development: Gradually add more complex parts of the code. Test each part as you go to catch errors early.
- Code Quality: Write clean, readable code. Use meaningful variable names, and add comments if necessary to explain complex parts.
4. Test and Debug
- Test Cases: Run your code with a variety of test cases, including typical cases, edge cases, and any tricky scenarios you identified.
- Debugging: If your code doesn’t work as expected, use debugging techniques to find and fix issues. Check for logical errors, syntax errors, and edge cases you might have missed.
- Optimize: Once your code works correctly, review it for potential optimizations. Consider time and space complexity and see if there’s a more efficient solution.